What is Binary Classification?
Binary Classification is a fundamental task in Machine Learning where the goal is to classify input data into one of two categories or classes. It's called "binary" because there are only two possible outcomes. For example, determining whether an email is spam or not spam, predicting whether a tumor is malignant or benign, or identifying whether a transaction is fraudulent or legitimate are all examples of Binary Classification problems.
How does Binary Classification work?
- Data Collection - You start with a dataset that contains labeled examples of input data along with their corresponding class labels. Each example consists of one or more features (also known as predictors or attributes) and a binary class label indicating the category it belongs to.
- Data Preprocessing - Before training a model, it's often necessary to preprocess the data. This may involve steps such as removing missing values, normalizing or scaling features, encoding categorical variables, and splitting the dataset into training and testing sets.
- Model Training - Once the data is preprocessed, you select a Machine Learning algorithm suitable for Binary Classification and train it using the training data. Popular algorithms for Binary Classification include logistic regression, decision trees, support vector machines (SVM), and neural networks.
- Model Evaluation - After training the model, you evaluate its performance using the testing data. Common evaluation metrics for Binary Classification include accuracy, precision, recall, F1 score, and area under the receiver operating characteristic curve (AUC-ROC).
- Model Tuning - Depending on the evaluation results, you may need to fine-tune the model by adjusting hyperparameters, trying different algorithms, or performing feature engineering to improve its performance.
- Prediction - Once you're satisfied with the model's performance, you can use it to make predictions on new, unseen data. The model takes the features of the input data as input and outputs a predicted class label (either 0 or 1, representing the two classes).
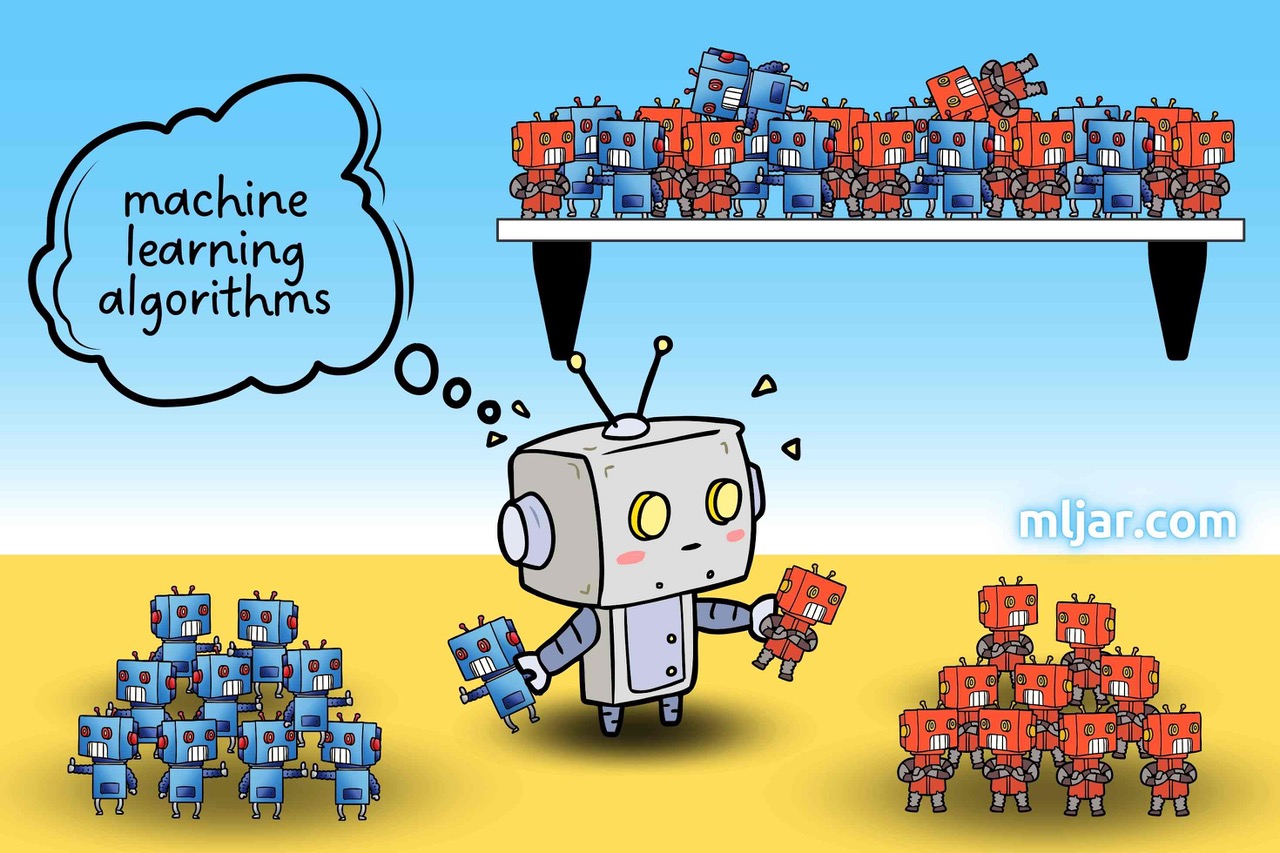
History of Binary Classification:
Binary Classification can trace its roots back to early statistical methods developed in the 20th century. Concepts such as linear regression and logistic regression, which were developed in the early 20th century, laid the foundation for Binary Classification. Logistic regression, in particular, is a key technique used for Binary Classification, as it models the probability of a binary outcome.
In the late 1950s and early 1960s, Frank Rosenblatt introduced the perceptron, a computational model inspired by the functioning of neurons in the brain. The perceptron could learn to classify input data into two categories by adjusting the weights of its connections based on feedback. While the perceptron was limited to linearly separable data, it laid the groundwork for more advanced neural network models.
Decision trees, which originated in the 1960s, are another important technique for Binary Classification. Decision trees recursively partition the feature space into regions based on feature values, leading to a tree-like structure where each leaf node represents a class label. Decision trees are intuitive, interpretable, and can handle both numerical and categorical data.
If ypu want to know more, read our entry about decision trees.
Binary Classification in Python:
# Import necessary libraries
import numpy as np
from sklearn.model_selection import train_test_split
from sklearn.linear_model import LogisticRegression
from sklearn.metrics import accuracy_score, classification_report
from sklearn.datasets import make_classification
# Generate synthetic Binary Classification data
X, y = make_classification(n_samples=1000, n_features=10, n_classes=2, random_state=42)
# Split the data into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Initialize and train a logistic regression classifier
clf = LogisticRegression()
clf.fit(X_train, y_train)
# Make predictions on the test set
y_pred = clf.predict(X_test)
# Evaluate the classifier
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy:", accuracy)
# Display classification report
print("Classification Report:")
print(classification_report(y_test, y_pred))
In this code:
- We import necessary libraries including numpy for numerical operations, scikit-learn for machine learning, and specifically LogisticRegression for Binary Classification.
- We generate synthetic Binary Classification data using make_classification() function from scikit-learn.
- The data is split into training and testing sets using train_test_split().
- A logistic regression classifier is initialized and trained on the training data using the fit() method.
- Predictions are made on the test set using the predict() method.
- We evaluate the classifier's performance using accuracy() and the classification_report(), which includes precision, recall, and F1-score for each class.
You can replace the synthetic data generation part with your own dataset and adapt the code accordingly based on your specific use case. Additionally, you can explore other classifiers and fine-tune hyperparameters to improve performance.
Binary Classification Use cases:
These are just a few examples, but Binary Classification is widely applicable across numerous domains, including fraud detection, sentiment analysis, fault diagnosis, and more. Its versatility and effectiveness make it a fundamental technique in the field of machine learning and data science.
-
Email Spam Detection:
- Binary Classification is commonly used in email systems to detect spam messages. The goal is to classify incoming emails as either spam or non-spam (ham). Features such as the email content, sender's address, subject line, and attachments can be used to train a classifier. Techniques like logistic regression, support vector machines, or even deep learning models can be employed for this task. By accurately identifying spam emails, email providers can protect users from phishing attempts, malware, and unwanted solicitations.
-
Medical Diagnosis:
- In medical diagnosis, Binary Classification is often used to distinguish between patients who have a particular medical condition and those who do not. For example, in breast cancer diagnosis, a Binary Classifier can be trained to classify mammogram images as either showing signs of malignancy or not. The classifier may use features extracted from the images, such as texture, shape, and density of abnormalities. Accurate classification can aid healthcare professionals in early detection and treatment planning, potentially saving lives.
-
Credit Scoring:
- Binary Classification is extensively employed in credit scoring to predict whether a loan applicant is likely to default on their loan or not. Lenders use various features such as credit history, income, employment status, and debt-to-income ratio to train a classifier. The classifier distinguishes between "good" applicants who are likely to repay their loans and "bad" applicants who are at risk of default. This helps financial institutions make informed decisions about extending credit, setting interest rates, and managing risk in their lending portfolios.
Pros and Cons:
Binary Classification offers several advantages and disadvantages:
-
Advantages:
- Simplicity - Binary Classification is conceptually simple compared to multi-class classification. With only two possible outcomes, it's easier to understand and implement.
- Efficiency - Binary Classification algorithms often require less computational resources compared to multi-class classification algorithms. This can result in faster training and inference times, making them suitable for large datasets and real-time applications.
- Interpretability - Binary Classification models are often more interpretable than their multi-class counterparts. For example, logistic regression models provide interpretable coefficients that indicate the impact of each feature on the classification decision.
-
Disadvantages:
- Limited Scope - Binary Classification is inherently limited to problems with only two possible outcomes. Some real-world scenarios may have more than two classes, requiring the use of multi-class classification techniques.
- Imbalanced Data - In many real-world applications, the classes may be imbalanced, meaning one class significantly outnumbers the other. Imbalanced data can lead to biased models that favor the majority class. Special techniques, such as resampling or cost-sensitive learning, may be necessary to address this issue.
- Decision Threshold - Binary Classifiers rely on a decision threshold to assign class labels. Choosing an appropriate threshold can be challenging and may depend on the specific requirements of the problem. Moreover, changing the threshold can trade off between precision and recall, requiring careful consideration.
Literature:
-
"Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" by Aurélien Géron - This practical guide covers a wide range of machine learning topics, including Binary Classification, with hands-on examples using popular Python libraries such as scikit-learn, Keras, and TensorFlow.
-
"Fast imbalanced Binary Classification: a moment-based approach" by Edouard Grave and Laurent El Ghaoui - This article explores the problem of imbalanced Binary Classification in which the number of negative examples is much larger than the number of positive examples.
-
"Pattern Recognition and Machine Learning" by Christopher M. Bishop - This book offers a comprehensive introduction to pattern recognition and machine learning algorithms, including Binary Classification methods such as logistic regression, support vector machines, and decision trees.
Conclusions:
Binary Classification is widely used in various applications across industries, including healthcare, finance, marketing, and cybersecurity, among others. It forms the basis for many more complex machine learning tasks and algorithms.
MLJAR Glossary
Learn more about data science world
- What is Artificial Intelligence?
- What is AutoML?
- What is Binary Classification?
- What is Business Intelligence?
- What is CatBoost?
- What is Clustering?
- What is Data Engineer?
- What is Data Science?
- What is DataFrame?
- What is Decision Tree?
- What is Ensemble Learning?
- What is Gradient Boosting Machine (GBM)?
- What is Hyperparameter Tuning?
- What is IPYNB?
- What is Jupyter Notebook?
- What is LightGBM?
- What is Machine Learning Pipeline?
- What is Machine Learning?
- What is Parquet File?
- What is Python Package Manager?
- What is Python Package?
- What is Python Pandas?
- What is Python Virtual Environment?
- What is Random Forest?
- What is Regression?
- What is SVM?
- What is Time Series Analysis?
- What is XGBoost?