How to authenticate Python to access Google Sheets with Service Account JSON credentials
When you need to access Google Sheets data from Python script you will need to prove that you have access to the resource. There are several options to authenticate to Google API, one of them is Service Account. We will show you how to get JSON file with credentials to access Google Sheets.
Steps
Creating JSON file with credentials to access Google Sheets API is fast and easy. It can be summarized with the following steps.
- Create a new project
- Enable Google Drive API and Google Sheets API
- Create Service Account credentials
- Download JSON file with credentials
The schema showing the concept of how does it work.
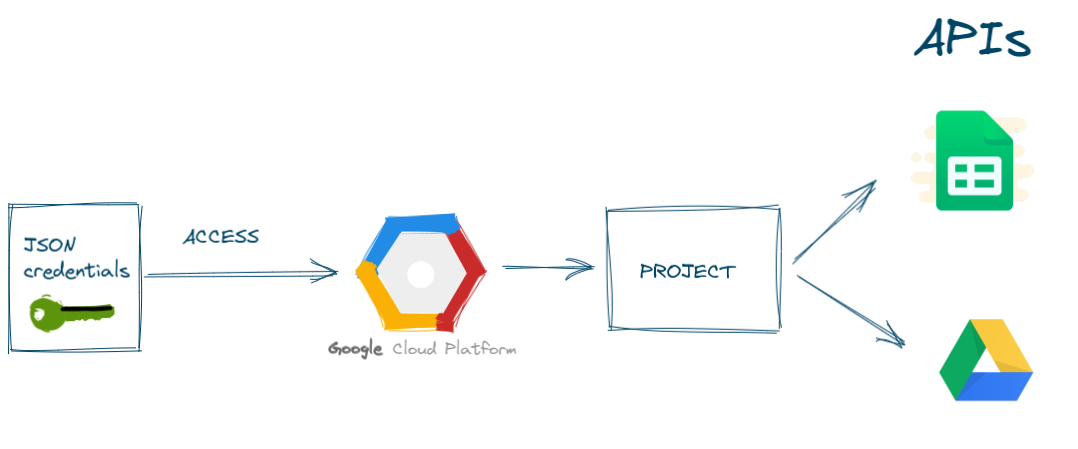
Create a new project
Please open your Google Cloud console in your web browser. You can open it by navigating the https://console.cloud.google.com/.
In this example, we will create a new project. It will store all details about our connection to Google Sheets API. In my opinion, it is good to have a separate project for accessing a Sheets, because in the case of emergency it is easy to revoke the access.
If it is your first project then you will see the view similar like in the image below:
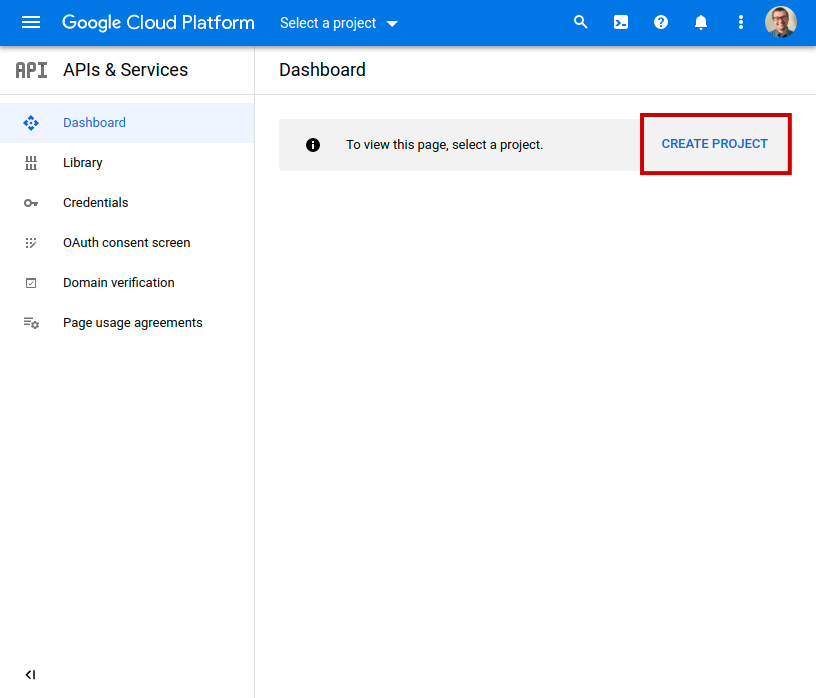
If you already have some projects in your console, please click on project in the top menu bar, and you will have dialog window with NEW PROJECT
button.
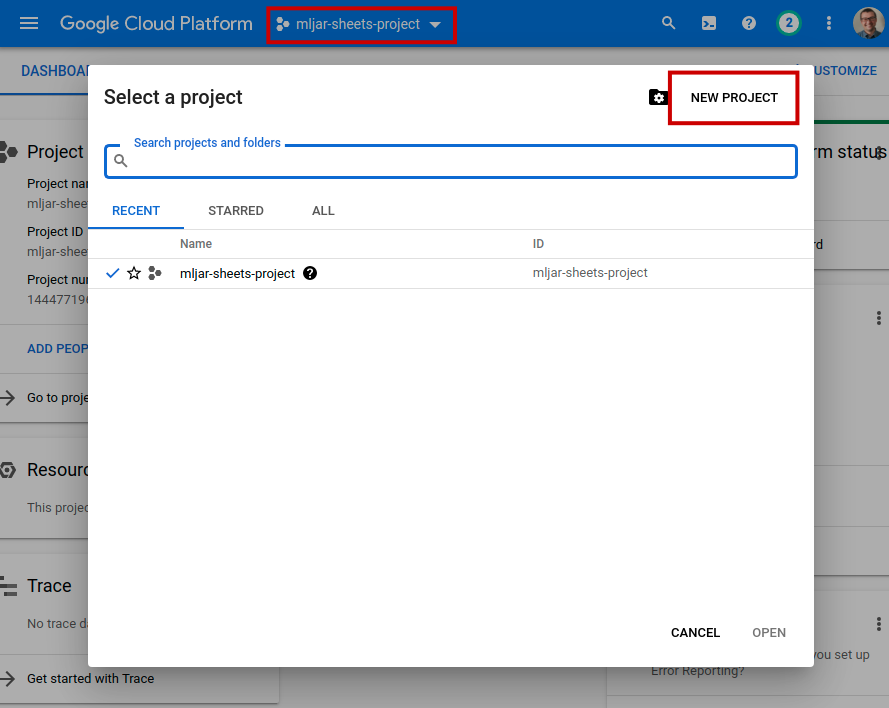
The form for project creation will be displayed. Just set the name and click CREATE
. In this example, I've used mljar-sheets-project
as a name. You can leave Location
field empty.
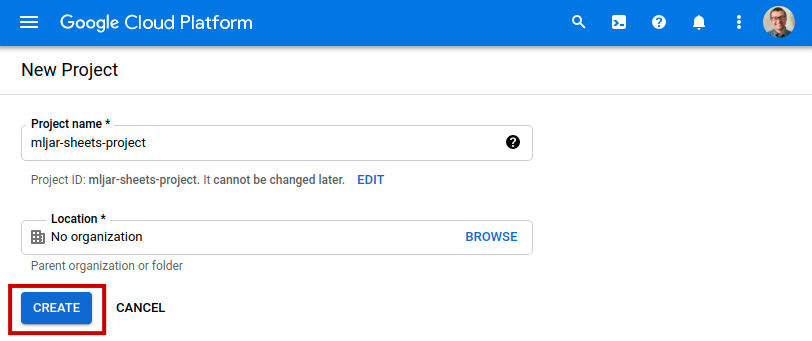
After Project creation, you should see view like in the image below. Please click ENABLE APIS AND SERVICES
button to add access to selected APIs.
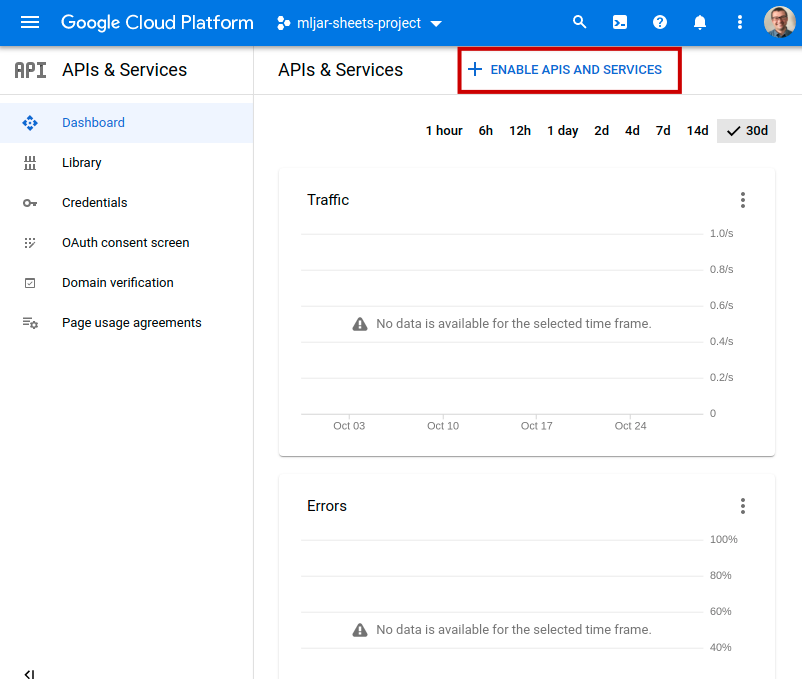
Enable access to APIs in project
In the next step, we will need to enable access to APIs for our project. The project will need to have access to:
- Google Drive API,
- Google Sheets API
Why do we need to give additional access to Google Drive API? It's because Google Sheets are stored in Google Drive. Changes made on Sheets are also changes on files in the Google Drive.
Let's search for Google Drive API
:
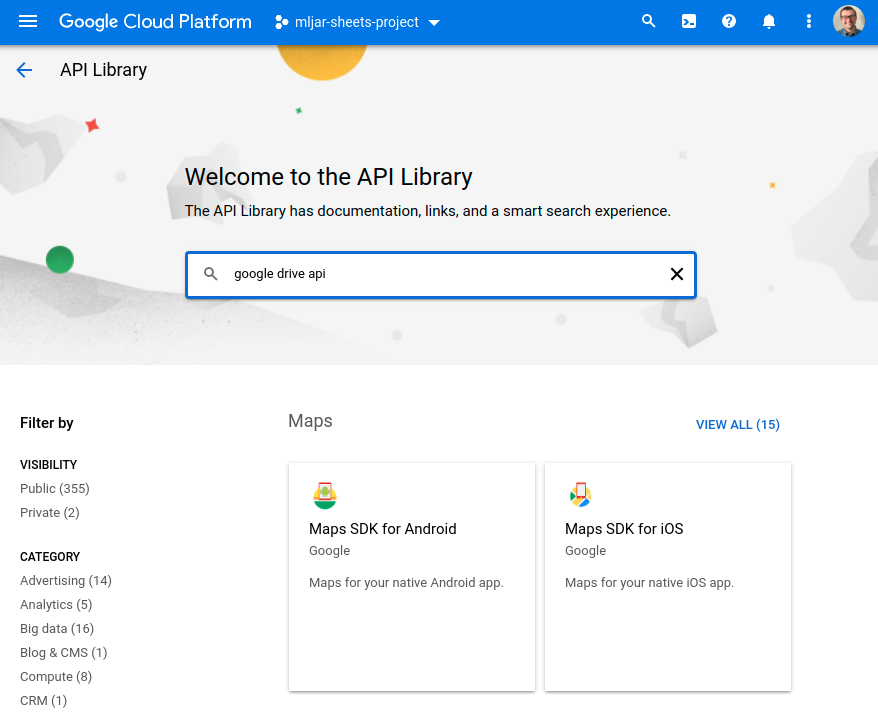
When you found it, just click ENABLE
button:
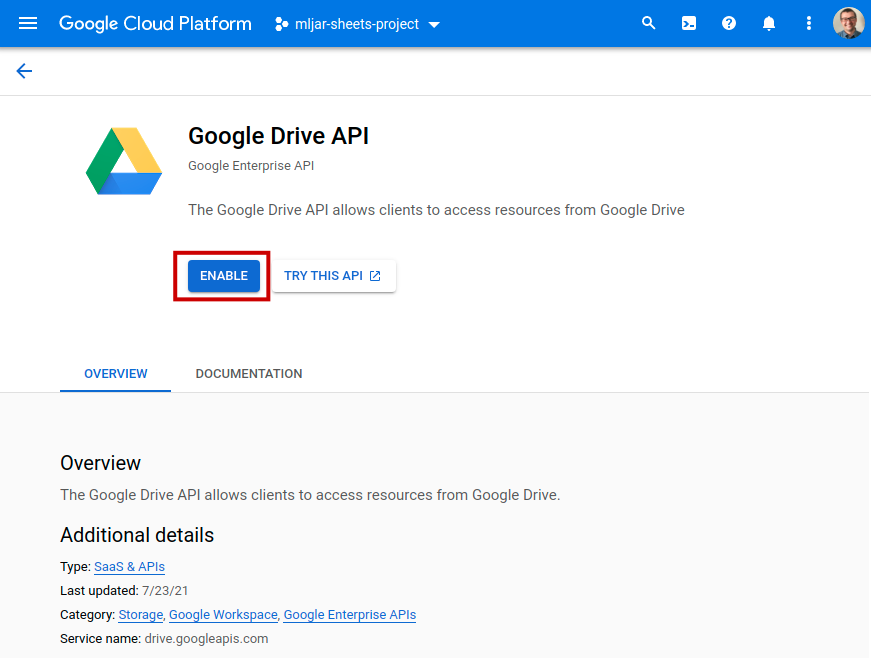
After API is enabled, you will see the below view. Please click on APIs & Service
in the left menu.
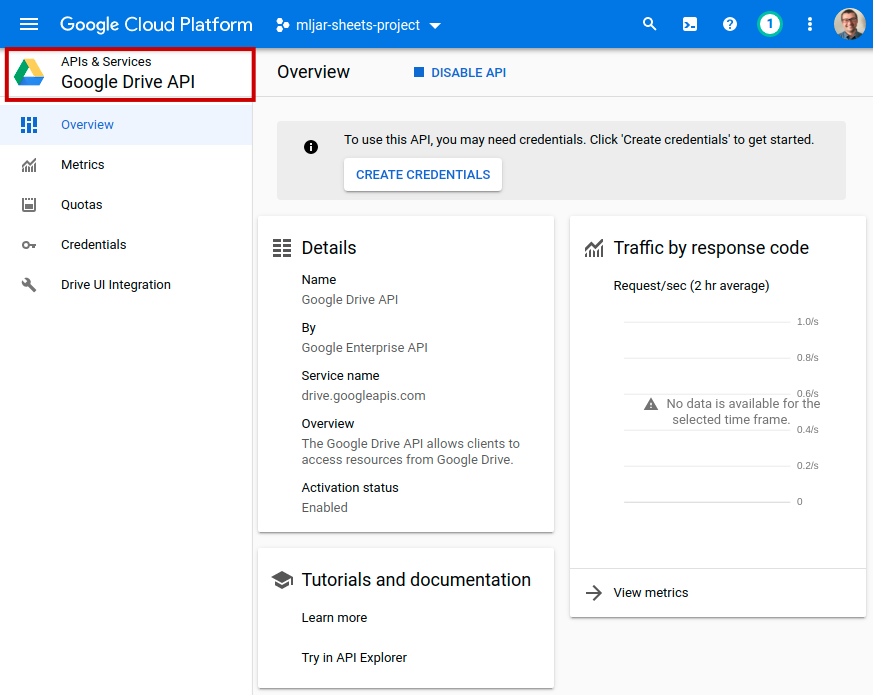
We need to search one more API. Please enter Google Sheets API
in the search box:
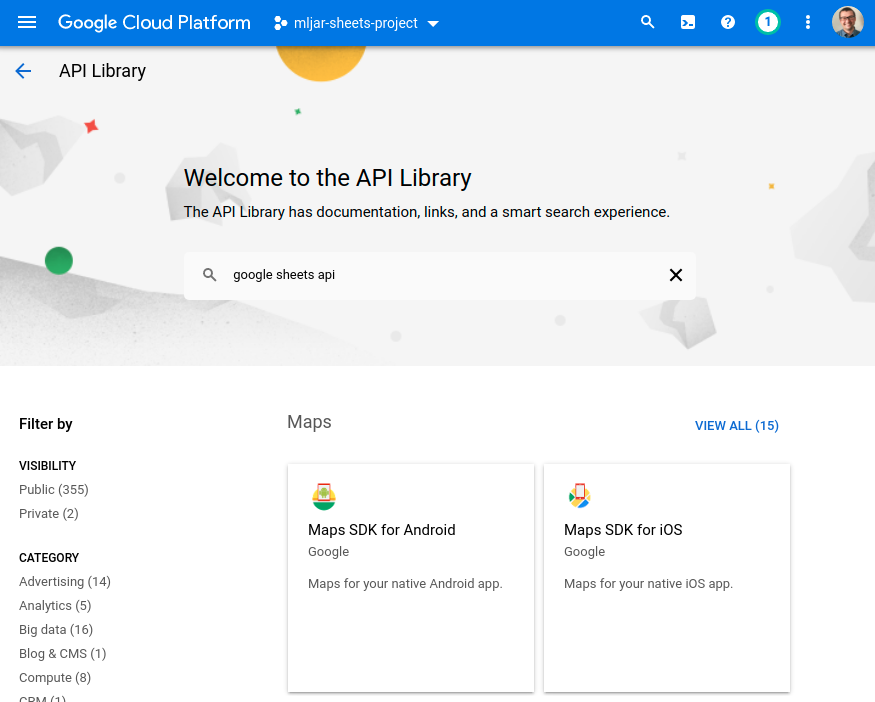
Like before, please enable it with ENABLE
button:
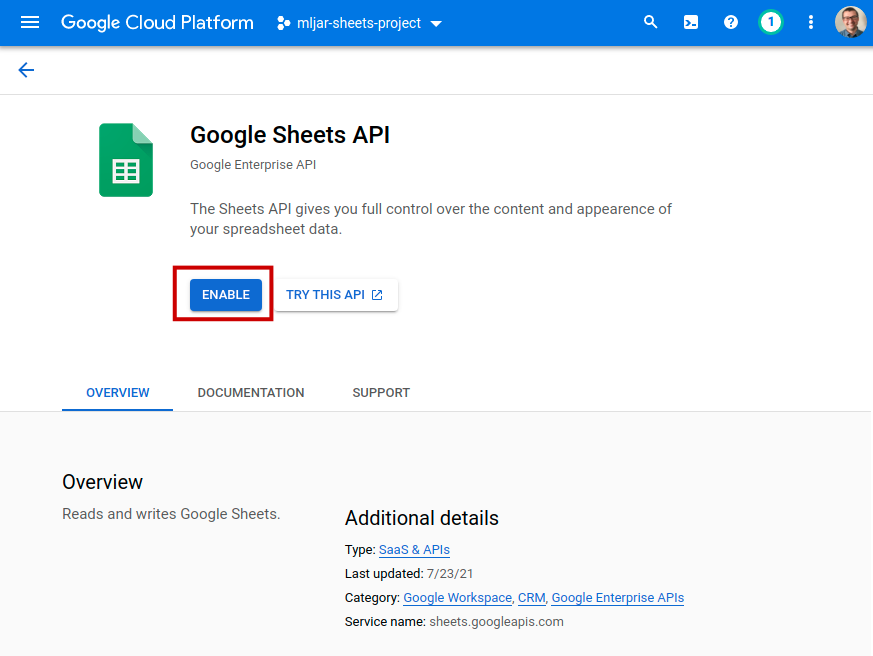
Create Service Account credentials
After enabling both (Drive and Sheets) APIs you will have the view like in the image below. You can click there CREATE CREDENTIALS
button.
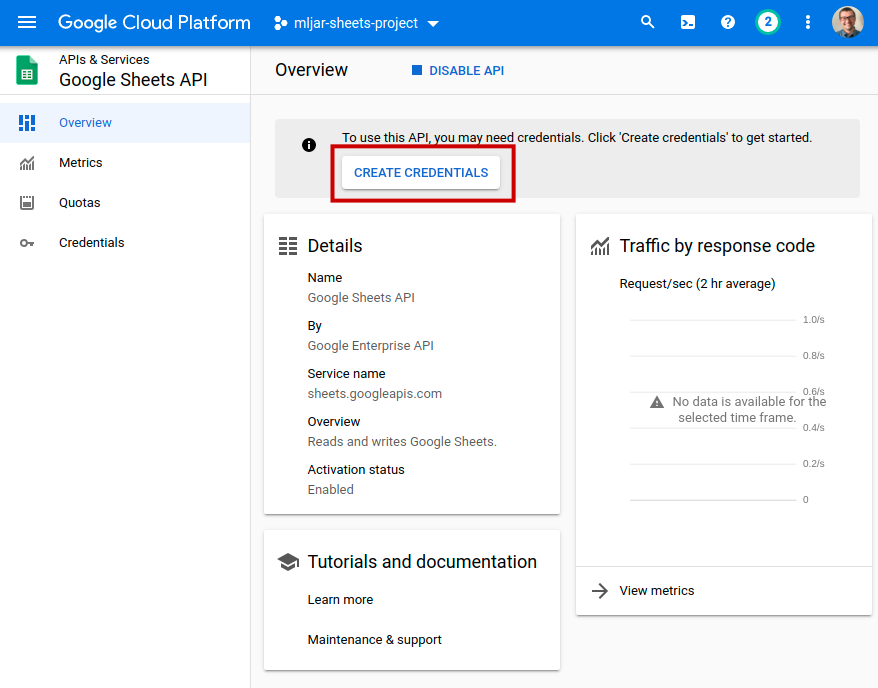
The other option for credentials creation is to go to the project dashboard and click CREATE CREDENTIALS
button there:
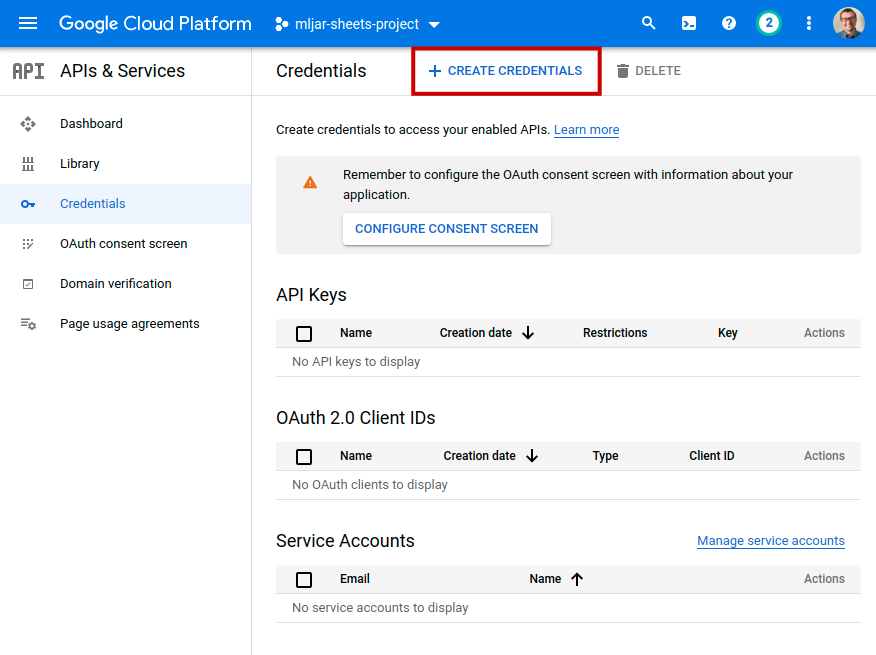
You will have a menu displayed with several options. Please select Service account
.
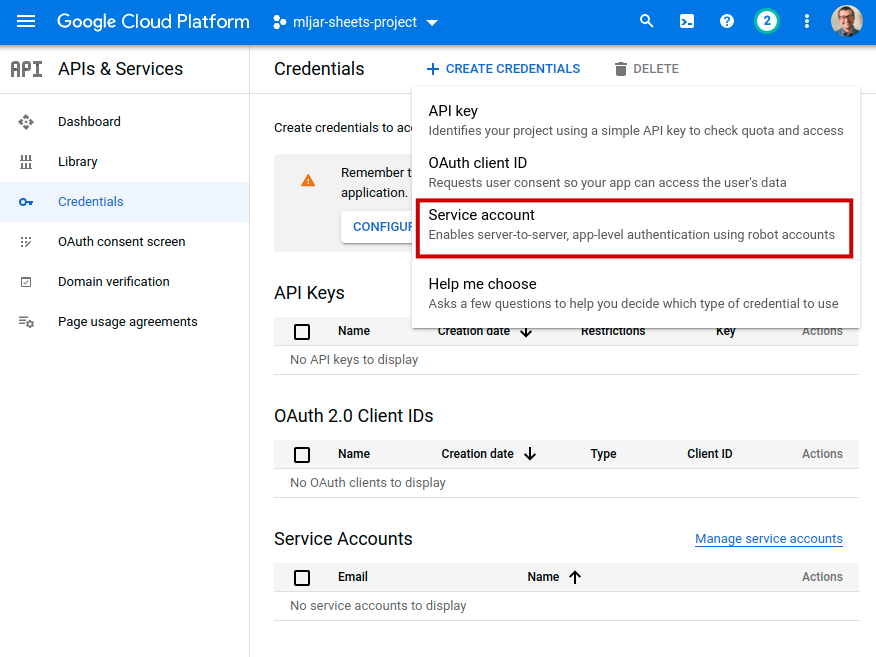
Service Account option will create a new account that we will use only to access Google Sheets API. Google Cloud will create a new email associated with Service Account. We will share selected Spreadsheets with that email. Such solution is good and safe in my opinion. We have an option to set a granular access to the resources that we want to share with our script. In the case of stolen credentials, we simply remove the credentials or even a whole project.
Please fill the Service account name and click CREATE AND CONTINE
.
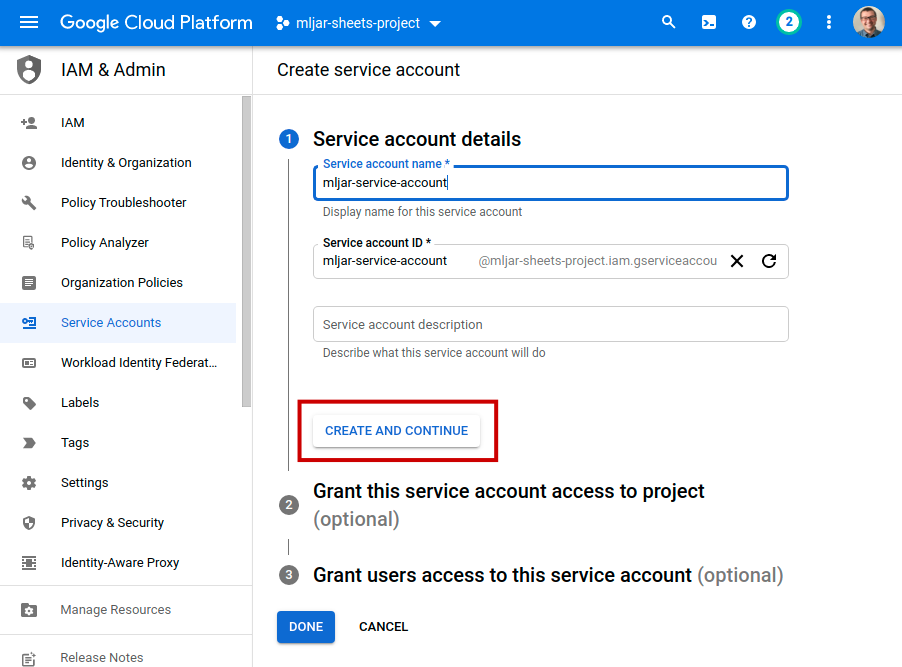
In the next step, set access to the project. In this example, I set the role to Owner
. You can set more restrictive role if needed. Click DONE
button.
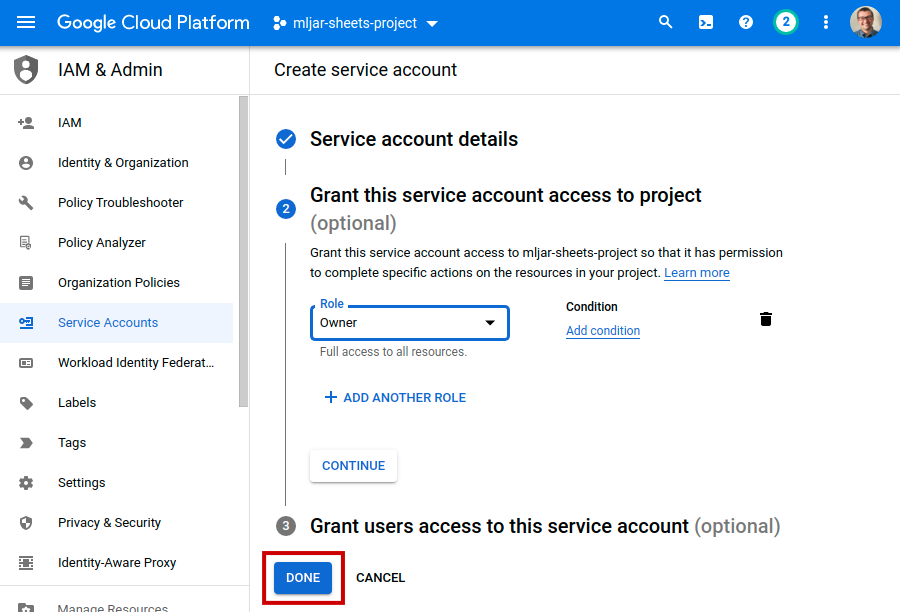
Create JSON file with credentials
After creating the Service Account you will see a view with your credentials. Please click on your Service Account.
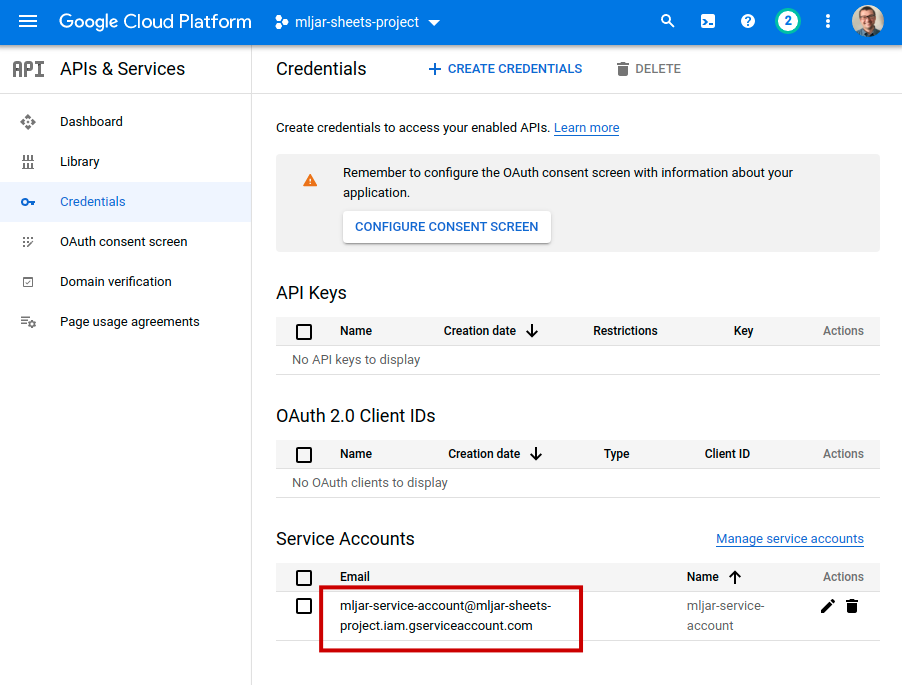
You will see its details. Please select KEYS
tab.
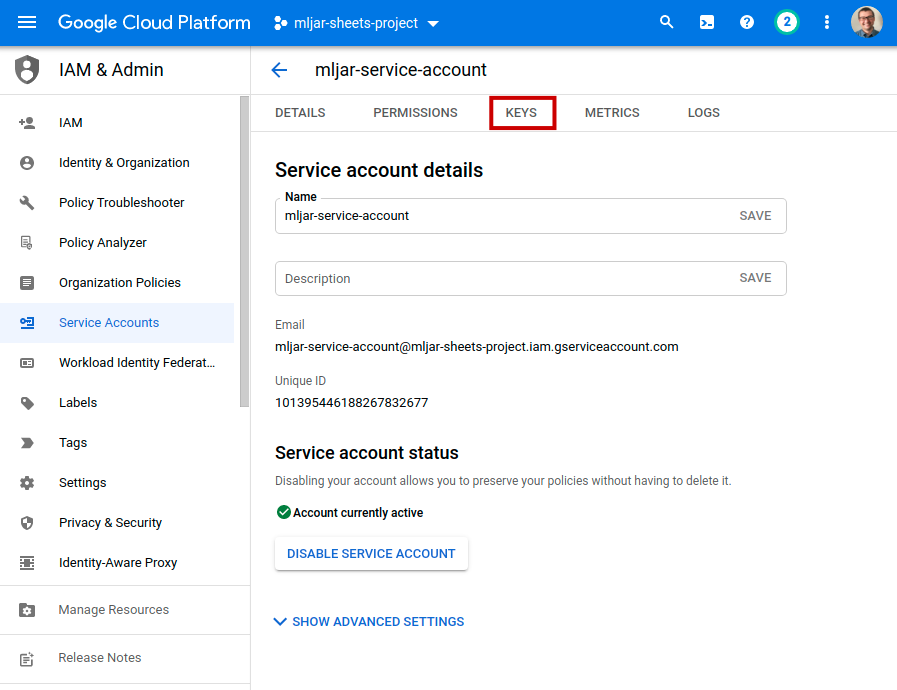
In the KEYS
view, just click ADD KEY
and Create new key
:
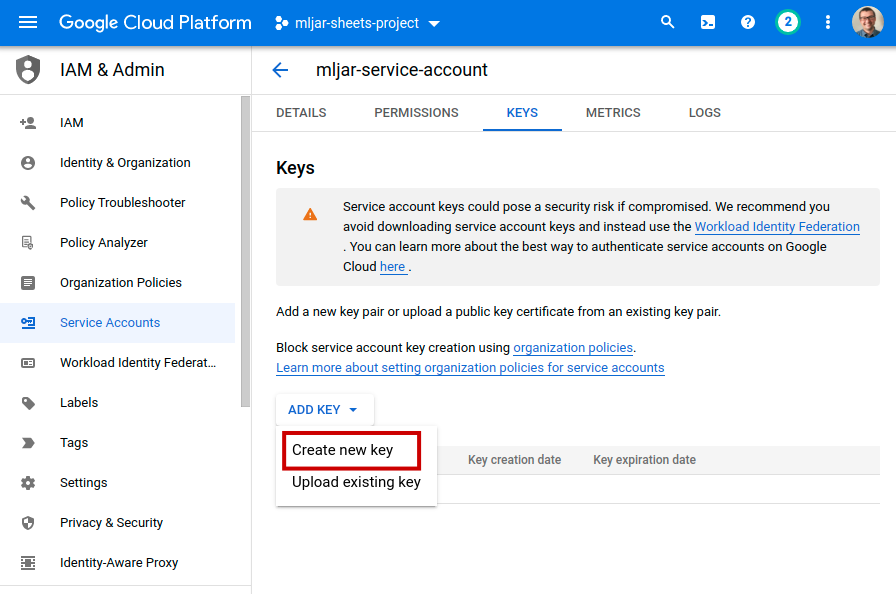
You will see a dialog where you can select the key format. Please select JSON (should be the default) and click CREATE
. It should start download of your JSON file with credentials.
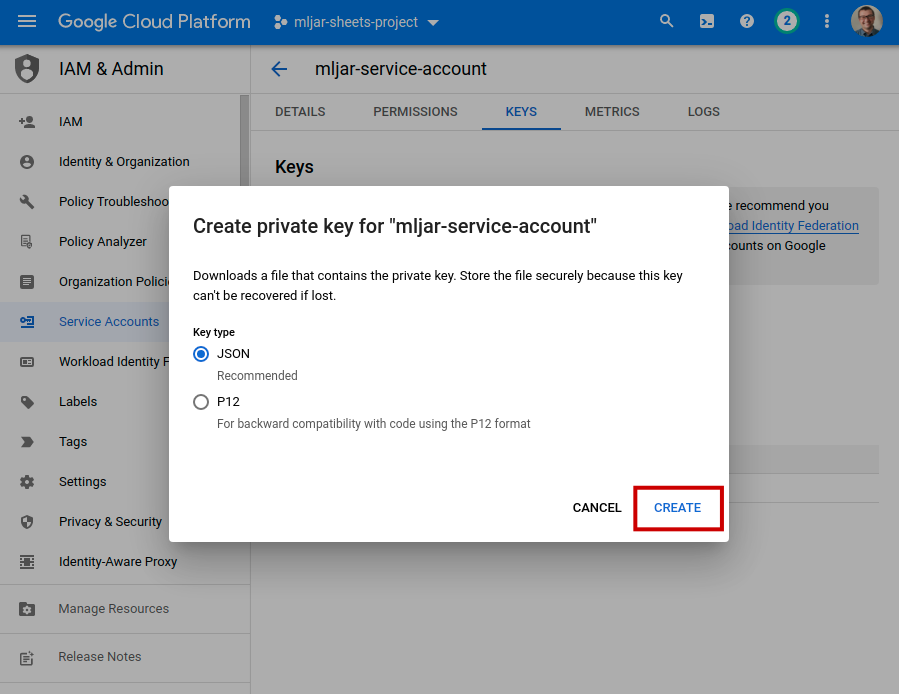
Congratulations! The JSON file is ready to use in your Python scripts. Let's test it.
Authenticate in Python
I will use Python code that is generated with MLJAR Studio notebook:
import gspread # connect got Google Sheets gspread_client = gspread.service_account(filename="/path/to/your/credentials.json") # list all available spreadsheets spreadsheets = gspread_client.openall() if spreadsheets: print("Available spreadsheets:") for spreadsheet in spreadsheets: print("Title:", spreadsheet.title, "URL:", spreadsheet.url) else: print("No spreadsheets available") print("Please share the spreadsheet with Service Account email") print(gspread_client.auth.signer_email)
This is how it looks in the MLJAR Studio:
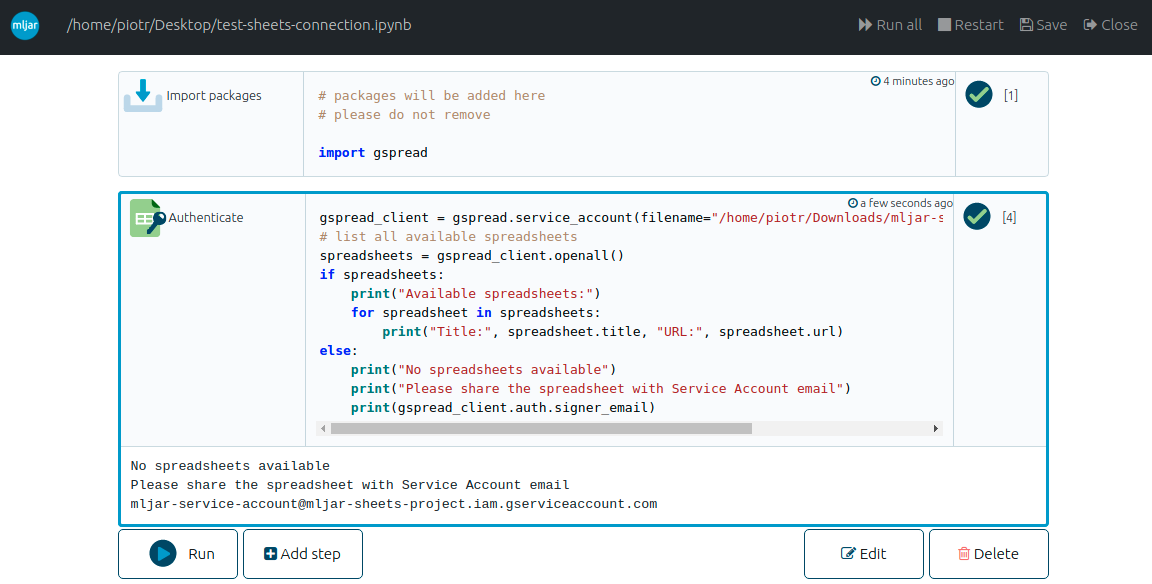
You should see the message that no spreadsheets are available. You will see also an email that is associated with Service account. You need to share Spreadsheets with that email.
The email can be also checked in Credentials
view in Google Cloud Console:
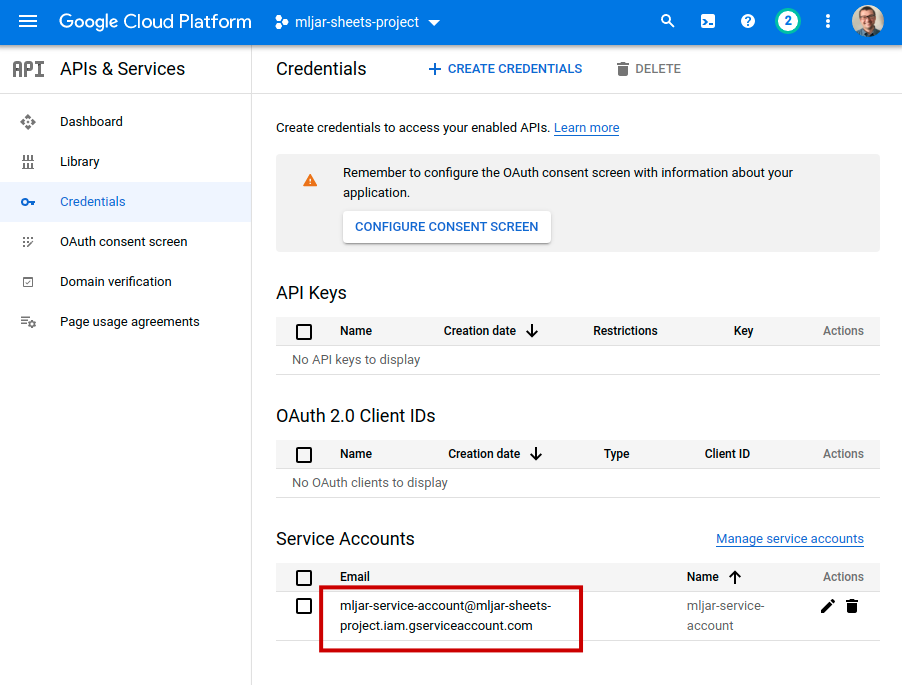
You share the Spreadsheet with Service Account email in the same way as you will share with a friend:
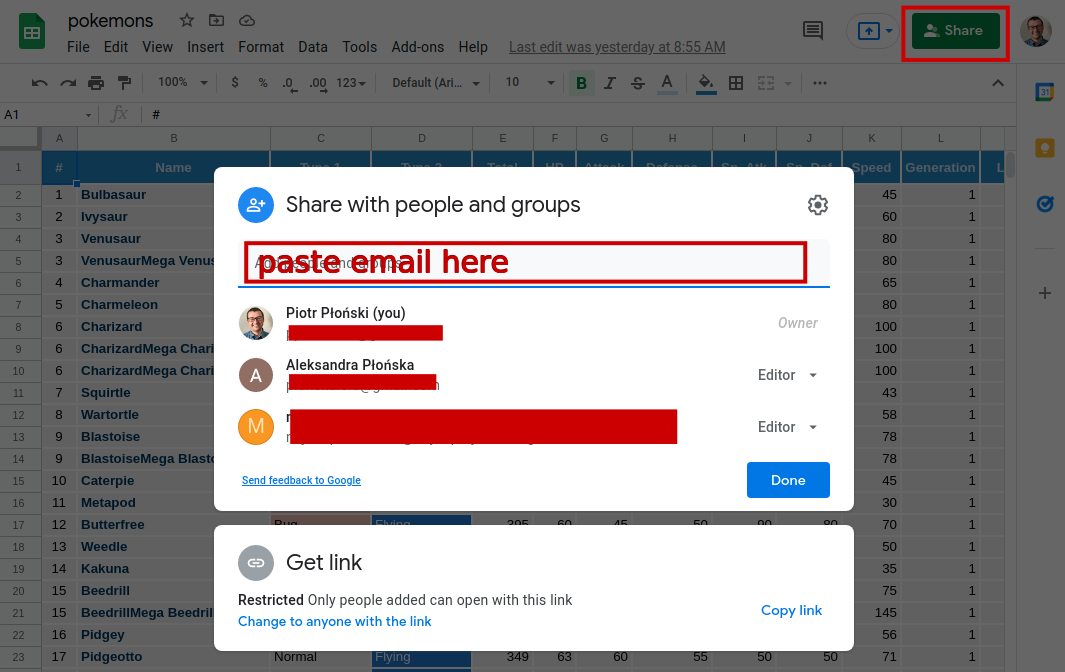
Remove the project
If you would like to remove the project, please click three dots near your profile picture in the top right corner and select Project settings
.
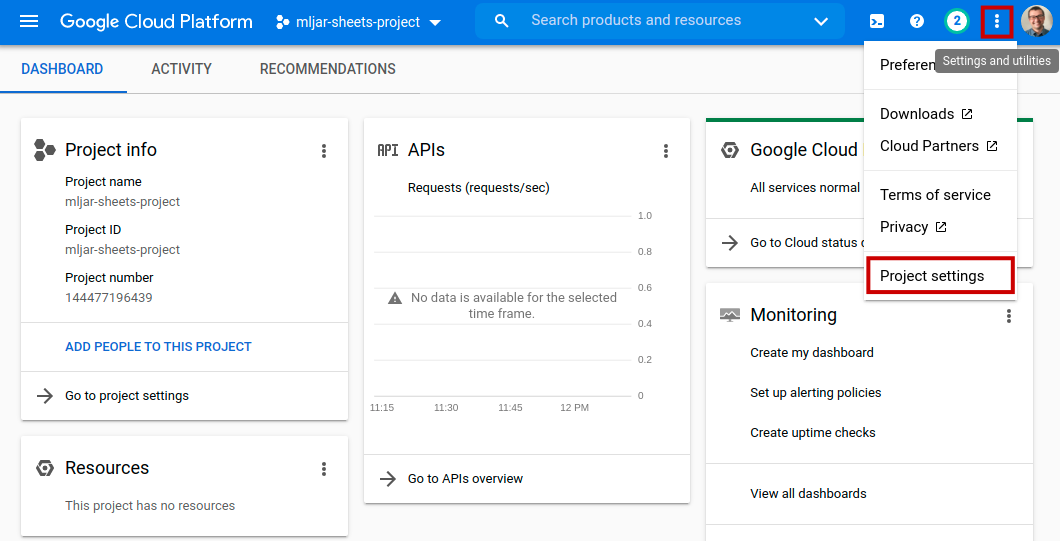
There, please click SHUT DOWN
to delete the project.
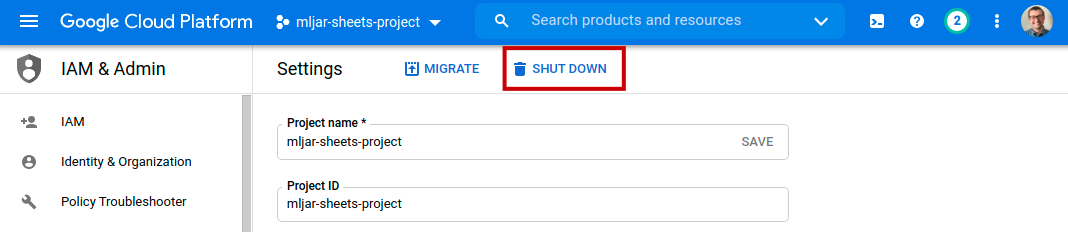
That'all, project is scheduled for removal.
Summary
In this article you have created a JSON file with credentials to authenticate Python code to access Google Sheets data. We use Service Account credentials. To have access to the selected Spreadsheet, it needs to be shared with email associated with Service Account. It is much safer apporach than sharing all your Spreadsheets at once.
We are looking for feedback or comments from you. You can reach us by email to Ola.
We are working on MLJAR Studio desktop application. It is a no-code framework for creating Python scripts. The application is still under the development. If you would like to be informed about the release please fill the form.
About the Authors
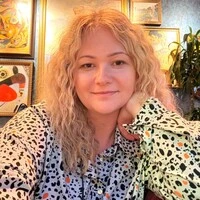
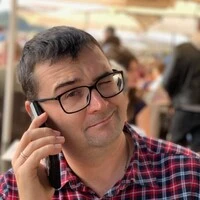
Related Articles
- How to use early stopping in Xgboost training?
- CatBoost with custom evaluation metric
- The next-generation of AutoML frameworks
- MLJAR Studio a new way to build data apps
- Read Google Sheets in Python with no-code MLJAR Studio
- Python Virtual Environment Explained
- Share Jupyter Notebook as web app
- File upload widget in Jupyter Notebook web app
- Build Computer Vision Web App with Python, OpenCV and Mercury
- Develop NLP Web App from Python Notebook