Develop NLP Web App from Python Notebook
Natural Language Processing (NLP) is a scientific field working on interactions between computers and human written language. The machines are programmed and taught to understand the text and extract information.
In this post, I will show you how to develop a web application for sentiment analysis. The end-user will be able to enter text, and the web app will compute its sentiment. In this article I will show you:
- how to create Python code for sentiment analysis with Jupyter Notebook, TextBlob and SpaCy,
- how to convert the notebook into a web application with Mercury framework,
- how to deploy the web app to Heroku (using free dyno).
The preview of the application is presented in the GIF below:

Looks interesting? Let's start - you will learn a lot! 🤓 📚
Setup a GitHub repository
Please create a new GitHub repository. After repository creation, please clone the repository.
git clone git@github.com:pplonski/nlp-apps-mercury.git
My repository is public and available on GitHub https://github.com/pplonski/nlp-apps-mercury.
Set virtual environment and install requirements
Before starting coding, let's create a virtual environment.
virtualenv nlpenv
source nlpenv/bin/activate
The nlpenv
is the name of my virtual environment. Please add requirements.txt
file with the needed packages:
mljar-mercury
spacy
spacytextblob
To install packages please run:
pip install -r requirements.txt
We will use en_core_web_sm
model from SpaCy. Please download it with the command:
python -m spacy download en_core_web_sm
To use the new virtual environment in the Jupyter run:
python -m ipykernel install --user --name=nlpenv
The above command creates the kernel with the same name as virtual environment.
Create a new Notebook
Please start Jupyter Notebook with the following command:
jupyter notebook
Let's create a new notebook. Remember to select a kernel with the name of the virtual environment nlpenv
. Set the notebook's name to the sentiment_analyzer.ipynb
.
We will import the required packages in the first cell:
import spacy
from IPython.display import Markdown as md
from spacytextblob.spacytextblob import SpacyTextBlob
In the second cell, let's define the input text. We will compute the sentiment for it.
# User input text
user_input = 'This is an amazing app'
Let's load SpaCy models:
# load NLP models
nlp = spacy.load('en_core_web_sm')
_=nlp.add_pipe('spacytextblob')
and process the input text to compute the sentiment:
# process user input and compute sentiment
doc = nlp(user_input)
The sentiment value is in a variable doc._.polarity
. Additionally, there are computed subjectivity and assessments. You can read more on spaCyTextBlob docs.
We can display the information about the sentiment as an alert block with the color corresponding to the sentiment value. The negative sentiment will be displayed as a danger alert (red color), the neutral will be a warning alert (yellow color), and the positive is a success alert (green). The sentiment is negative when the polarity
is lower than -0.5
. The sentiment is positive when polarity
is higher than 0.5
. The display code is below:
emojis = ["😠", "😐", "😊"]
headers = ["Negative", "Neutral", "Positive"]
alerts = ["danger", "warning", "success"]
index = 1
if doc._.polarity >= 0.5:
index = 2
elif doc._.polarity <= -0.5:
index = 0
# display the alert
md(f'''
<div class="alert alert-block alert-{alerts[index]}">
<h2>{emojis[index]} {headers[index]} sentiment</h2>
<h3>{user_input}</h3>
<h4>Polarity: {doc._.polarity}</h4>
</div>
''')
The full notebook's code:
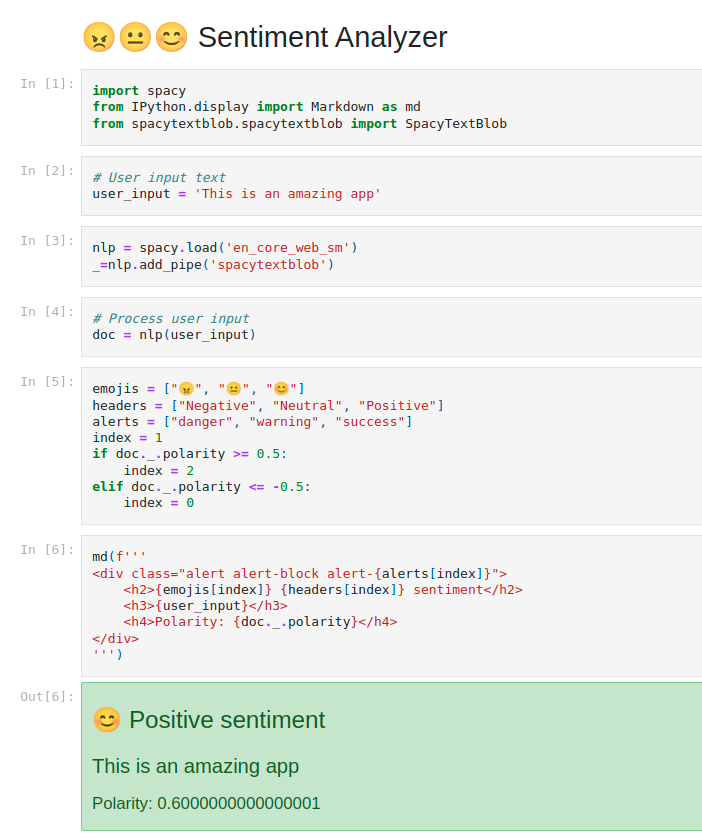
If you want to share this notebook with friends, they will need to install all required packages and download the SpaCy model. Then they will need to change the user_input
variable and re-run the whole notebook. It is not a very convenient way of notebook sharing. The Mercury framework is solving this problem.
Share notebook as web app with Mercury
You can easily transform your notebook into a web app. The user input will be a text widget. The user will be able to enter the text and click the Run
button to execute the notebook with new values. Adding widgets to the notebook is as easy as adding a new cell. Please add a new RAW cell at the top of the notebook. We will add a YAML header there:
title: 😠😐😊 Sentiment Analyzer
description: Enter text to get its sentiment
show-code: False
params:
user_input:
input: text
rows: 5
label: Please enter text
value: This is an amazing app
In the three first lines we define the title
of the app, the description
, and hide the notebook's code (show-code: False
). In the params
there is one variable. It is called user_input
. We define it as a text input with 5 rows.
That's all! The notebook can be used as a web application. Let's see what it looks like locally. Please run the following command:
mercury watch sentiment_analyzer.ipynb
At the http://127.0.0.1:8000 address, you should have the Mercury application running - please check your website. The sentiment analyzer app should like in the screenshot below:
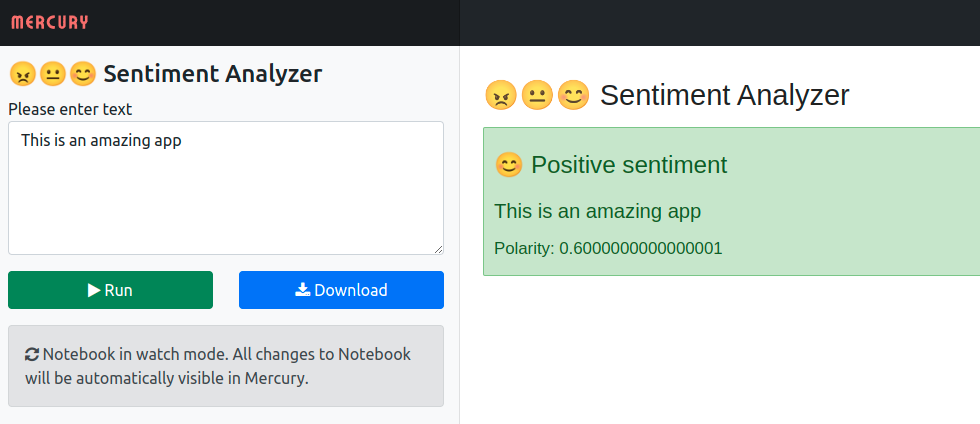
Please notice that you will see all the notebook's live updates when running Mercury locally and watching the notebook.
Deploy to Heroku
The Mercury framework can be deployed to the server on any cloud. In this post, I'm using Heroku because they provide free dyno - perfect for creating small, demo apps. Let's create a new Heroku app (I assume you have Heroku CLI tools installed):
heroku create nlp-apps-mercury
We will need to add Procfile
that will tell Heroku how to run our app. The Procfile
file contents:
web: python -m spacy download en_core_web_sm && mercury run 0.0.0.0:$PORT
Please notice that in the Procfile
we first download the SpaCy model and then run the Mercury server & worker at 0.0.0.0:$PORT
address.
Deploy with one command:
git push heroku main
You should wait a while (about 1 minute), and the app should be running in the cloud. ☁️☁️☁️ My app is running at https://nlp-apps-mercury.herokuapp.com/.
Conclusions
You can build a sentiment analyzer notebook with Python. A package like SpaCy and TextBlob makes it easy. However, there is a problem when you would like to share your Python notebook with others, especially non-programmers. The Mercury can help with your work sharing. You can add interactive widgets to the notebook by simply inserting a YAML configuration in the first raw cell. The framework will serve the notebook as a web application. It can be deployed to any server.
- The NLP web app repo 👉 https://github.com/pplonski/nlp-apps-mercury
- The NLP web app running at Heroku 👉 https://nlp-apps-mercury.herokuapp.com/
- Mercury GitHub repo 👉 https://github.com/mljar/mercury