Save a Plot to a File in Matplotlib (using 14 formats)
The Matplotlib
is a popular plotting library for Python. It can be used in Python scripts and Jupyter Notebooks. The plot can be displayed in a separate window or a notebook. What if you would like to save the plot to a file? In this article, I will show you how to save the Matplotlib
plot into a file. It can be done by using 14 different formats.
Quick code snippet
Below is a quick Python code snippet that shows how to save a figure to a file.
import matplotlib.pyplot as plt # create a plot plt.plot([4,2,3,1,5]) # save figure plt.savefig("my_plot.png")
Important:
- Please call
plt.show()
afterplt.savefig()
. Otherwise, you will get an empty plot in the file. - For Jupyter Notebook users, you need to call
plt.savefig()
in the same cell in which the plot is created. Otherwise, the file has an empty figure.
The savefig
function - deep dive
All plots created with Matplotlib
are saved with savefig
function. Please take a while a look into savefig
documentation.
Below are savefig
arguments that you might find useful:
fname
- a path where to save a plot. It can be without extension. Forfname
with the extension included theformat
will be automatically selected. Common extensions:.png
,.pdf
,.svg
;dpi
- a numeric value for dots per inch; it controls the size of the figure in pixels. You can read more in an article on how to change figure size inMatplotlib
;bbox_inches
- controls the white space around the figure, if you don't need margins, please set this parameter totight
;transparent
- is a boolean value that can turn the plot background into transparent;format
- is a string with the output file format. Common formats arepng
,pdf
,svg
. If not specified, it is automatically set from thefname
extension. The default ispng
.
You can list all available formats in Matplotlib
with below Python code:
import matplotlib.pyplot as plt print(plt.gcf().canvas.get_supported_filetypes())
Output:
{
'eps': 'Encapsulated Postscript',
'jpg': 'Joint Photographic Experts Group',
'jpeg': 'Joint Photographic Experts Group',
'pdf': 'Portable Document Format',
'pgf': 'PGF code for LaTeX',
'png': 'Portable Network Graphics',
'ps': 'Postscript',
'raw': 'Raw RGBA bitmap',
'rgba': 'Raw RGBA bitmap',
'svg': 'Scalable Vector Graphics',
'svgz': 'Scalable Vector Graphics',
'tif': 'Tagged Image File Format',
'tiff': 'Tagged Image File Format'
}
There are, in total 13 formats supported by Matplotlib
.
Example of advanced save
Let's try to save a figure to PNG format with transparent background with a size of 1200x800
pixels:
import matplotlib.pyplot as plt plt.figure(figsize=(6,4), dpi=200) plt.plot([4,2,3,1,5]) plt.savefig("my_plot.png", transparent=True)
We set the size of the figure during creation, the size in pixels is computed by multiplying figsize
by dpi
.
Save Matplotlib
figure
Earlier, we save plots in graphics formats. What if we would like to save the whole Matplotlib
figure for further manipulation? It is possible. We can use the pickle
library. It is a Python object serialization package. It doesn't need to be installed additionally.
Here is an example Python code that creates the Matplitlib
figure, saves to a file, and loads it back:
import pickle import matplotlib.pyplot as plt # create figure fig = plt.figure() plt.plot([4,2,3,1,5]) # save whole figure pickle.dump(fig, open("figure.pickle", "wb")) # load figure from file fig = pickle.load(open("figure.pickle", "rb"))
Summary
You can save the Matplotlib
plot to a file using 13 graphics formats. The most common formats are .png
, .pdf
, .svg
and .jpg
. The savefig()
function has useful arguments: transparent
, bbox_inches
, or format
. There is an option to serialize the figure object to a file and load it back with the pickle
package. It can be used to save the figure for future manipulations.
About the Authors
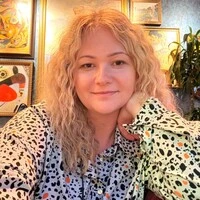
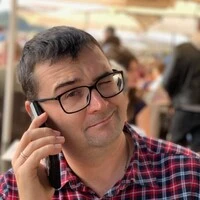
Related Articles
- The 3 ways to share password protected Jupyter Notebook
- The 3 ways to change figure size in Matplotlib
- The 5 ways to publish Jupyter Notebook Presentation
- The 2 ways to save and load scikit-learn model
- Complete list of 594 PyTZ timezones
- Convert Jupyter Notebook to Python script in 3 ways
- 3 ways to get Pandas DataFrame row count
- 9 ways to set colors in Matplotlib
- Jupyter Notebook in 4 flavors
- Python Dashboard for 15,963 Data Analyst job listings