What is DataFrame?
A DataFrame is a fundamental data structure in the pandas library, which is widely used for data manipulation and analysis in Python. It represents a tabular, spreadsheet-like data structure containing rows and columns, where each column can have a different data type (e.g., integer, float, string).
DataFrames are highly flexible and allow for various operations such as filtering, selecting, aggregating, merging, and reshaping data. They provide powerful tools for cleaning and analyzing data, making them a popular choice for working with structured data in Python.
Advantages:
DataFrame's popularity stems from several key factors:
1. Ease of Use:
DataFrame provides a simple and intuitive interface for handling structured data, making it accessible to both beginners and experienced users. Its syntax is designed to be concise and readable, reducing the amount of code required for common data manipulation tasks.
2. Flexibility:
DataFrame offers a wide range of functionalities for data manipulation, including filtering, selecting, aggregating, merging, and reshaping data. It supports various input and output formats, allowing users to work with data from different sources seamlessly.
3. Performance:
DataFrame is by default built on top of NumPy, a high-performance numerical computing library in Python. As a result, it leverages NumPy's optimized data structures and operations, leading to efficient computation even with large datasets.
4. Integration:
DataFrame integrates well with other libraries and tools commonly used in the Python ecosystem, such as NumPy
, Matplotlib
, scikit-learn
, and more. This interoperability enables users to combine DataFrame with other tools for advanced data analysis, visualization, and machine learning tasks.
5. Community Support:
pandas, the library that provides DataFrame functionality, has a large and active community of users and contributors. This community provides extensive documentation, tutorials, and online resources, making it easier for users to learn and troubleshoot issues.
6. Industry Adoption:
DataFrame has gained widespread adoption across industries, including finance, healthcare, technology, and academia. Its versatility and robustness have made it a go-to tool for data analysis and manipulation in various domains.
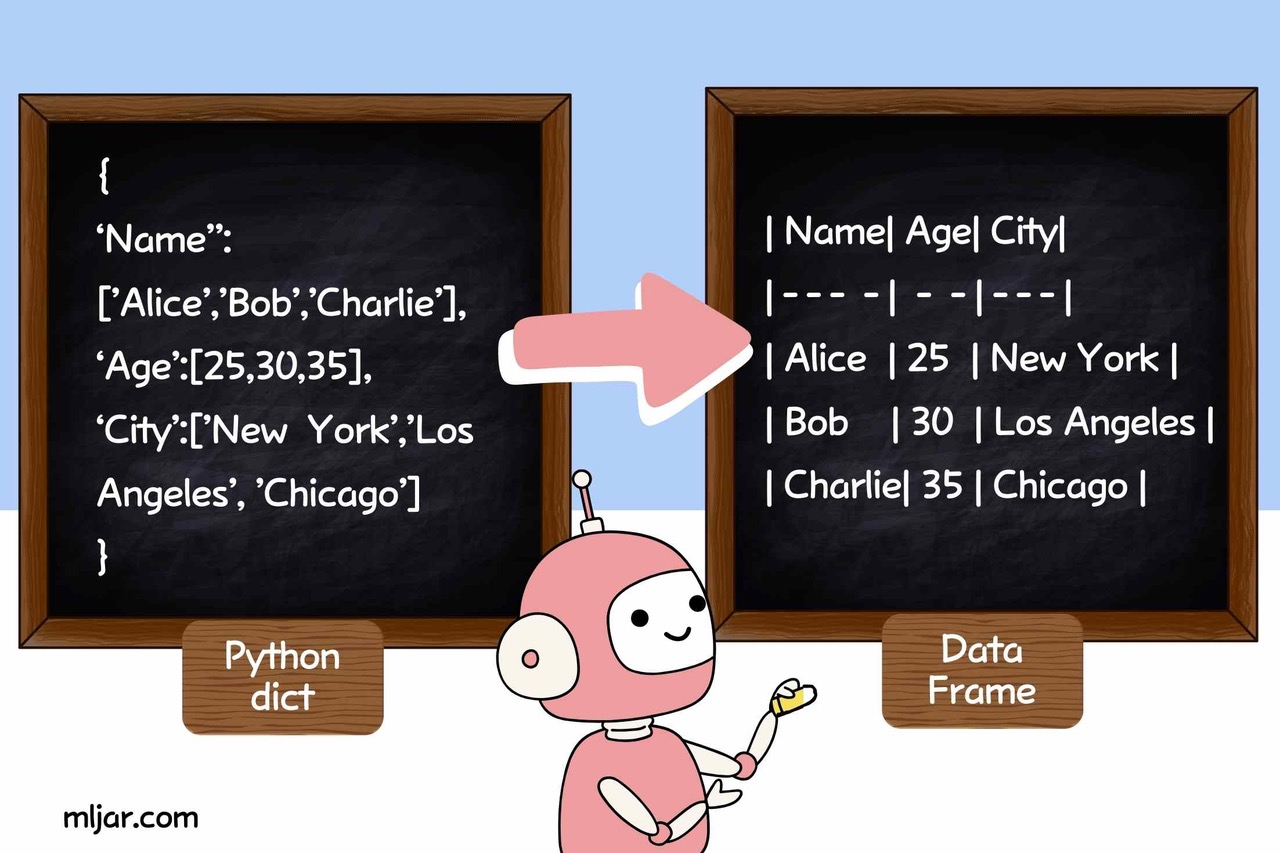
Other Vendors:
Pandas is the most widely used library for working with DataFrames in Python, but there are other libraries and tools that offer similar functionality. Some of these alternatives include:
-
Dask: Dask is a parallel computing library that extends the functionality of pandas to larger-than-memory or distributed datasets. It provides DataFrame and Series structures that mimic pandas' interface while allowing for parallel and distributed computation.
-
Vaex: Vaex is a high-performance Python library for lazy, out-of-core DataFrames. It is designed to work efficiently with large datasets that do not fit into memory by utilizing memory-mapped files. Vaex aims to provide a pandas-like API with better performance for large-scale data analysis.
-
Modin: Modin is a library that accelerates pandas by using parallel and distributed computing. It allows users to switch between pandas' single-threaded mode and Modin's parallel mode seamlessly, providing faster computation for DataFrame operations.
-
cuDF (GPU-accelerated DataFrames): cuDF is part of the RAPIDS suite of open-source libraries for GPU-accelerated data science and machine learning. It provides a GPU-accelerated DataFrame library that is compatible with pandas, allowing users to leverage the power of NVIDIA GPUs for faster data processing.
-
Spark DataFrame: Apache Spark is a distributed computing framework that offers a DataFrame API for working with structured data. Spark DataFrames provide similar functionality to pandas DataFrames but are designed to scale out across a cluster of machines for processing large-scale datasets.
While pandas remains the most commonly used library for working with DataFrames in Python, these alternatives offer specialized features and performance enhancements for specific use cases, such as handling larger datasets, parallel processing, or GPU acceleration. Depending on your requirements and constraints, you may choose one of these alternatives over pandas.
Most DataFrame libraries, such as Dask, Vaex, and Modin, strive to maintain compatibility with pandas' syntax and interface. This means that basic operations and methods should work similarly across these libraries. It has to be remembered, while some DataFrames may look simmilar, there can be differences in their behavior, performance, and supported features, making those DataFrames noninterchangeable.
Using DataFrame in Python:
Sure, here are the steps involved in the provided code:
1. Import pandas:
Import the pandas library, usually aliased as pd
, to utilize its DataFrame functionality.
import pandas as pd
2. Create DataFrame from dictionary:
Define a dictionary containing the data and pass it to the pd.DataFrame()
function to create a DataFrame.
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Emma'],
'Age': [25, 30, 35, 40, 45],
'City': ['New York', 'Los Angeles', 'Chicago', 'Houston', 'Miami'],
'Salary': [60000, 70000, 80000, 90000, 100000]
}
df = pd.DataFrame(data)
3. Display DataFrame:
Print the DataFrame to the console to visualize its contents.
print("Original DataFrame:")
print(df)
Output:
Original DataFrame:
Name Age City Salary
0 Alice 25 New York 60000
1 Bob 30 Los Angeles 70000
2 Charlie 35 Chicago 80000
3 David 40 Houston 90000
4 Emma 45 Miami 100000
4. Get DataFrame information:
Use info()
method to display basic information about the DataFrame, such as column names, data types, and memory usage.
print("Information about the DataFrame:")
print(df.info())
Output:
Information about the DataFrame:
<class 'pandas.core.frame.DataFrame'>
RangeIndex: 5 entries, 0 to 4
Data columns (total 4 columns):
# Column Non-Null Count Dtype
--- ------ -------------- -----
0 Name 5 non-null object
1 Age 5 non-null int64
2 City 5 non-null object
3 Salary 5 non-null int64
dtypes: int64(2), object(2)
memory usage: 288.0+ bytes
None
5. Summary statistics:
Utilize describe()
method to compute summary statistics (count, mean, standard deviation, min, quartiles, max) for numerical columns.
print("Summary statistics of numerical columns:")
print(df.describe())
Output:
Summary statistics of numerical columns:
Age Salary
count 5.000000 5.000000
mean 35.000000 80000.000000
std 7.905694 15811.388301
min 25.000000 60000.000000
25% 30.000000 70000.000000
50% 35.000000 80000.000000
75% 40.000000 90000.000000
max 45.000000 100000.000000
6. Select specific columns:
Use indexing to select specific columns from the DataFrame.
print("Selecting specific columns:")
print(df[['Name', 'Age']])
Output:
Selecting specific columns:
Name Age
0 Alice 25
1 Bob 30
2 Charlie 35
3 David 40
4 Emma 45
7. Filter rows based on condition:
Apply boolean indexing to filter rows based on a condition.
print("Filtering rows based on a condition:")
print(df[df['Age'] > 30])
Output:
Filtering rows based on a condition:
Name Age City Salary
2 Charlie 35 Chicago 80000
3 David 40 Houston 90000
4 Emma 45 Miami 100000
8. Sort DataFrame:
Use sort_values()
method to sort the DataFrame by a column.
print("Sorting the DataFrame by Age:")
print(df.sort_values(by='Age'))
Output:
Sorting the DataFrame by Age:
Name Age City Salary
0 Alice 25 New York 60000
1 Bob 30 Los Angeles 70000
2 Charlie 35 Chicago 80000
3 David 40 Houston 90000
4 Emma 45 Miami 100000
9. Add new column:
Assign a list or array to a new column name to add it to the DataFrame.
df['Gender'] = ['Female', 'Male', 'Male', 'Male', 'Female']
print("DataFrame after adding a new column:")
print(df)
Output:
DataFrame after adding a new column:
Name Age City Salary Gender
0 Alice 25 New York 60000 Female
1 Bob 30 Los Angeles 70000 Male
2 Charlie 35 Chicago 80000 Male
3 David 40 Houston 90000 Male
4 Emma 45 Miami 100000 Female
10. Grouping and aggregation:
Use groupby()
method to group data by a column and apply an aggregation function (e.g., mean) to compute statistics for each group.
print("Grouping data by Gender and computing mean Age:")
print(df.groupby('Gender')['Age'].mean())
Output:
Grouping data by Gender and computing mean Age:
Gender
Female 35
Male 35
Name: Age, dtype: int64
These steps illustrate various operations that can be performed on a DataFrame using pandas in Python.
Literature:
You can learn more about DataFrames from various resources available online. Here are some suggestions:
-
Pandas Documentation: The official documentation for the pandas library provides comprehensive guides, tutorials, and examples covering all aspects of DataFrames.
-
"Python for Data Analysis" by Wes McKinney - Authored by the creator of pandas, this book offers a detailed exploration of pandas and DataFrames, along with practical examples and case studies.
-
"Pandas Cookbook" by Theodore Petrou - This book provides a collection of recipes for common data manipulation tasks using pandas, including working with DataFrames.
-
If you're interested in alternative DataFrame libraries like Dask, Vaex, Modin, or cuDF, explore their official documentation and resources for learning more about their features and usage.
Conclusions:
The subject of DataFrames encompasses various aspects related to working with structured data in Python, primarily facilitated by the pandas library.
Overall, DataFrames are a powerful tool for working with structured data in Python, offering flexibility, ease of use, and a wide range of functionalities for data manipulation and analysis. Whether using pandas or alternative libraries, understanding DataFrames is crucial for effective data processing and analysis in Python.